Unity Vector3.Lerp vs Vector3.Slerp
There’s a lot of confusion on the differences between Vector3.Lerp and Vector3.Slerp. I know because I have been confused by it for a while. Even when I thought I had it covered (and I posted about it before) I was corrected to the reality that I wasn’t. The fact is, after you understand it, it’s quite simple.
Both Vector3.Lerp and Vector3.Slerp were created to be able to progress a Vector3 from a Start vector to an End vector. The easiest thing to think about is when you want to change the position of an object smoothly between a initial position to a final position. Let’s see how both functions work for the same scenario.
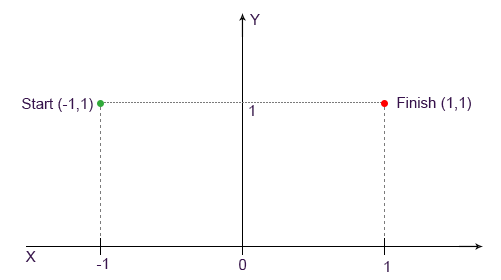
For the purpose of simplification we’re using 2D vectors, but it’s exactly the same behavior with 3D vectors.
Vector3.Lerp
Lerp will do a linear interpolation of the path from Start to Finish. You progress in the path by defining an interpolation amount “t” that varies from 0 (start) to 1 (finish).
public static Vector3 Lerp(Vector3 a, Vector3 b, float t);
Since it’s linear, if we input a t = 0.5f that means we’re halfway from Start to Finish. The same way as t = 0.1f we’re 10% of the way, and so on.
Let’s see a visual representation of several “t”s in the using our scenario
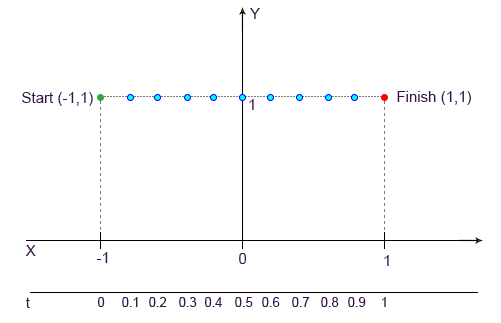
We now see the progression of the position from Start to Finish. This shows that the path is linear as is the relation between space traveled and the progression of “t”.
The real life use of Lerp in Unity could look something like this:
void Update() { t = (Time.time - startTime)/tripTime; transform.position = Vector3.Lerp(startPosition, endPosition, t); }
In this case we make t depend on the time. We can define how much time we want the whole trip to take (tripTime) and change the t on update accordingly.
Vector3.Slerp
Now let’s look at Vector3.Slerp. It’s a bit trickier to understand than Vector3.Lerp. Although it has the same method signature (same parameters) the result might be a bit different depending on where Start and Finish are.
public static Vector3 Slerp(Vector3 a, Vector3 b, float t);
While Lerp would give us a vector that is situated on the direct line between Start and Finish, Slerp will now treat the vector not as a position but as a direction. That means that we’re interpolating between two vector’s angle to the origin point and their radius.
Let’s see what happens if we do the same example but with Slerp.
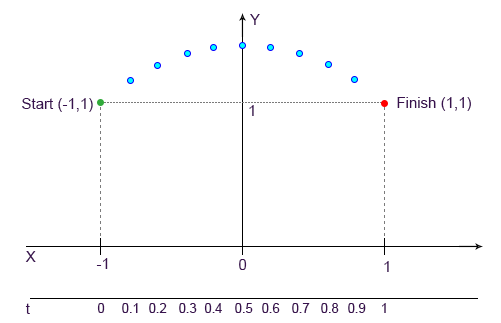
So now, instead of interpolating the positions between Start and Finish, we’re considering that Start and Finish are 2 vectors pointing out of the origin point (0,0) to different directions (angles). We know interpolate a vector between them that is pointing out of the origin in a direction between both. In this case, since both Start and Finish have the same distance to the origin, the interpolating vector has the same (radius) and all we’re actually changing is the angle.
Finally we can see a functioning example (if your browser supports WebGL) where you can see both functions in action.